How does pass updating work on Apple Wallet?
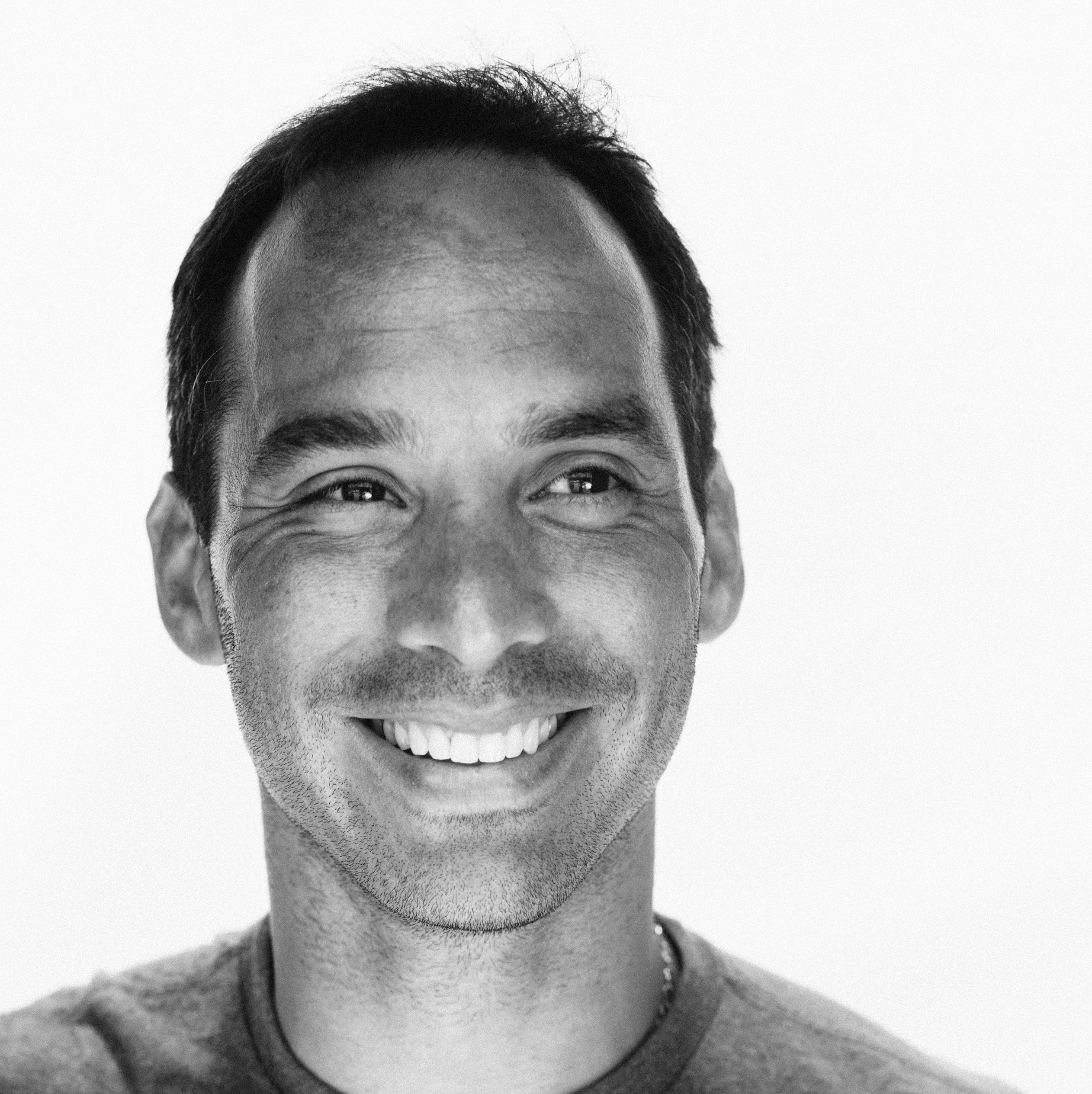
Updated October 17, 2024 03:29
Overview
This tutorial is designed to help developers quickly understand how to make passes in Apple wallet updatable. This means we will be able to edit the information on a pass that is already installed on an iPhone or Apple Watch and potentially generate a push notification in the process. Whether you're a seasoned developer or a beginner, this tutorial will get you through the steps like a ninja.
We will start by going over the steps to ensure your passes are compatible with updates, then we'll cover the device to server architecture and finally the steps to update a pass that has been installed.
This tutorial assumes that you:
- Have created a Pass type ID (tutorial here if needed)
- Understand how to issue and install passes on iOS devices
What makes a pass updatable in Apple Wallet
The first thing to know is that if you issue a pass that does not have the right configuration before install, you can never update that pass once it is installed. So this means that you must set proper configurations in your pass.json
file before the user downloads and installs your pkpass file. Which configurations exactly? Specifically you need to have webServiceURL
and authenticationToken
keys set in the top-level of your pass.json
file. Here is an abbreviated example:
{
"webServiceURL": "https://yourwebsite.com/",
"authenticationToken": "5up3r-53cr3t-t0k3n"
}
If you don't do this, your passes will not be updatable. This is the only way. If you have a pass that has been installed and it does not have these keys, then it is not updatable.
Device to server communications for updates
Once your passes have these configs set, you need to understand why they're important and how they're used. Apple has a comprehensive guide on how this works so we will only be going over the high level to save you time. The lifecycle basically goes like this:
- User installs pass
- Pass makes a call to register for updates at predetermined URL using domain listed above
- Server must record device details and return 200 HTTP response
- Something happens that requires the pass to be updated
- You send a request to Apple's APNS to let the iOS device know there has been an update
- The device makes a request to your servers for a list of updated passes
- Your server responds with the passes to be updated
- The device downloads and installs those passes automatically and provides a notification to the user
So when a pass is installed, the device sends a POST to a very specific URL, which looks like this:
https://yourwebsite.com/v1/devices/ef8662202e0292071caf7f56d881ed39/registrations/com.example.pass/12345
So you need to set up some code to support device registration. You'll get a JSON payload that looks a bit like this:
{
"pushToken": "406a05c6-86c3-469c-bda3-34571869f6b7"
}
Pretty simple, but you have to save all of this info to your database and protect that URL with authentication. The device will send an
Authorization
header with a value that looks likeApplePass 5up3r-53cr3t-t0k3n
- you'll need to make sure you don't save data that is not authenticated.
So, you've managed to handle pass update registrations! Next you need to support another endpoint that tells the device which passes have been updated since it last checked with you (which could be never). This URL looks like this:
https://yourwebsite.com/v1/devices/ef8662202e0292071caf7f56d881ed39/registrations/com.example.pass?passesUpdatedSince={previousLastUpdated}
This URL should find all of the passes that have been updated since the pass was last downloaded (which is passesUpdatedSince
). You need to return a JSON response that looks like this:
{
"serialNumbers": ["12345"],
"lastUpdated": "2024-02-09 01:28:49 UTC"
}
Where the serialNumbers array is a list of the pass IDs that should be updated and the most recent time of one these passes was updated. Additionally Apple likes for your response to have a last-modified
header that matches the lastUpdated
value.
Now that we have these two endpoints cooked up, we're ready.
Updating a pass that is already installed
So assuming you have set up the endpoints above correctly, have figured out how to issue a pass and you have saved the data from registrations. You're ready to send silent push notifications to the phone to prompt it to download the latest version of a pass, using the above endpoints, of course.
So for that, you'll need to integrate with APNS. There are a bunch of good libraries for this:
After you've set that up, you simply need to send Apple a notification that the pass is updated, using the pushToken
from the registration process. This prompts the device with the installed pass to query your update list endpoint, and then download the latest pass from the following URL:
https://yourwebsite.com/v1/passes/com.example.pass/12345
Then you must serve a valid and up-to-date pkpass file.
Updating Apple Wallet passes is easy with PassNinja
Dealing with certificates, pass type ids, creating custom endpoints, generating encryption keys, and more is very annoying for some people who want to just code.
That's why we created PassNinja! With our service, you create a custom API to meet your needs, use our ready-made SDKs in your favorite language, and just send an update call - it could literally be this simple:
import passninja
account_id = '**your-account-id**'
api_key = '**your-api-key**'
pass_ninja_client = passninja.PassNinjaClient(account_id, api_key)
simple_pass_object = pass_ninja_client.passes.put(
'ptk_0x14', # passType
'pid_NDQzXzQzMzQzMw==', # passId aka serialNumber
{
'discount': '100%',
'memberName': 'Ted'
} # pass object
)
print(simple_pass_object)
If you're ready to move fast and ship product, go sign up. It's free to get started, just click the "Get Started for Free" button in the top right of this website and fill out the form - we'll give you $10 in free credits!
Conclusion
We hope this tutorial demystified the process of updating passes in Apple Wallet, offering a step-by-step guide that covers initial configurations, server-side setup, and integration with APNS for push notifications. Additionally, the introduction of PassNinja showcases an innovative solution for developers seeking to simplify and accelerate the development of updatable passes for Apple Wallet.
If you have any feedback on this guide, please do not hesitate to reach out!
More articles focused on Apple Platform
In this article, we'll go over what happens when you create a pass type ID, issue passes using th...
How To Create Apple Pass Type IdsThis guide is designed to help developers quickly generate pass types on Apple's Developer portal...
How To Create Apple Wallet Nfc Encryption KeysThis guide is designed to help developers quickly generate an encryption keys for NFC enabled App...